Mastering Python Argparse Boolean Flags
Ever felt overwhelmed by complex command-line arguments in your Python scripts? Managing various options and parameters can quickly become a headache without the right tools. Enter `argparse`, a powerful Python module that simplifies the process of creating user-friendly command-line interfaces. One of its most useful features is the boolean flag, a simple yet effective way to toggle specific behaviors within your program.
Python's `argparse` module allows you to define flags that can be either set (True) or unset (False) by the user directly from the command line. This eliminates the need for complex logic to interpret different input values. Instead, you can focus on the core functionality of your script, leaving argument handling to `argparse`.
`argparse` boolean flags offer an elegant solution for managing optional features or settings within your applications. They are especially valuable when designing scripts intended for diverse use cases, as they provide a straightforward mechanism for users to customize behavior without requiring code modifications.
Imagine you're building a web scraper. You might want to include an option for verbose logging, enabling detailed output only when needed. A boolean flag like `--verbose` or `-v` makes this incredibly simple to implement. Users can activate verbose logging by including the flag when running the script and disable it by omitting it.
The concept of boolean flags in command-line tools isn't unique to Python. It's a common pattern found in various programming languages and operating system commands. The consistent use of boolean flags across different platforms contributes to a more intuitive user experience, enabling developers to leverage existing knowledge and expectations when designing their tools.
The `argparse` module became part of the Python standard library starting with Python 2.7 and 3.2, replacing the older `optparse` module. It provides a more flexible and robust approach to argument parsing. The main issue related to boolean flags, and more generally with command-line arguments, is ensuring clear documentation so users understand their purpose and usage.
To create a boolean flag using `argparse`, you use the `add_argument()` method of an `ArgumentParser` object. You specify the flag name (preceded by dashes, e.g., `--verbose`, `-v`) and use the `store_true` or `store_false` action to control the flag's behavior.
Example:
parser = argparse.ArgumentParser() parser.add_argument("--verbose", action="store_true", help="Enable verbose logging") args = parser.parse_args() if args.verbose: print("Verbose logging enabled")import argparse
Benefits of Using Boolean Flags:
1. Simplicity: They provide a clear and concise way to activate or deactivate features.
2. Flexibility: Easily combine multiple flags for granular control over script behavior.
3. User-Friendliness: Make your scripts easier to use by offering intuitive options.
Best Practices:
1. Use descriptive flag names.
2. Provide clear help messages.
3. Group related flags.
4. Use `store_true` for enabling features and `store_false` for disabling.
5. Consider using short and long versions of flags (e.g., `-v` and `--verbose`).FAQ:
1. What is the difference between `store_true` and `store_false`?
2. How can I combine multiple boolean flags?
3. Can I set a default value for a boolean flag?
4. How do I handle boolean flags with `argparse` in Python 2.7?
5. Can I use a different prefix other than `--` or `-` for boolean flags?
6. How do I create mutually exclusive boolean flags?
7. Can I use boolean flags with subcommands?
8. How can I access the value of a boolean flag within my script?Tips and Tricks:
Use the `default` argument in `add_argument()` to set an initial value for your boolean flag.
In conclusion, effectively using `argparse` boolean flags empowers you to create flexible and user-friendly Python scripts. The simplicity and clarity they offer make them an invaluable tool for managing optional behavior, simplifying complex command-line interfaces, and ultimately enhancing the overall user experience. By following best practices, understanding the nuances of `store_true` and `store_false`, and exploring the additional capabilities of `argparse`, you can create robust and adaptable scripts tailored to a wide range of needs. Take the time to master this powerful feature, and you'll find your Python scripting workflow greatly improved. Start incorporating boolean flags into your projects today to experience the benefits firsthand. Learn more by exploring the official Python `argparse` documentation and searching online tutorials for more advanced techniques.
Unlocking the secrets of sherwin williams revere pewter dupes
Sweet good morning messages to win their heart
Decoding optum rx part d coverage your prescription for savings









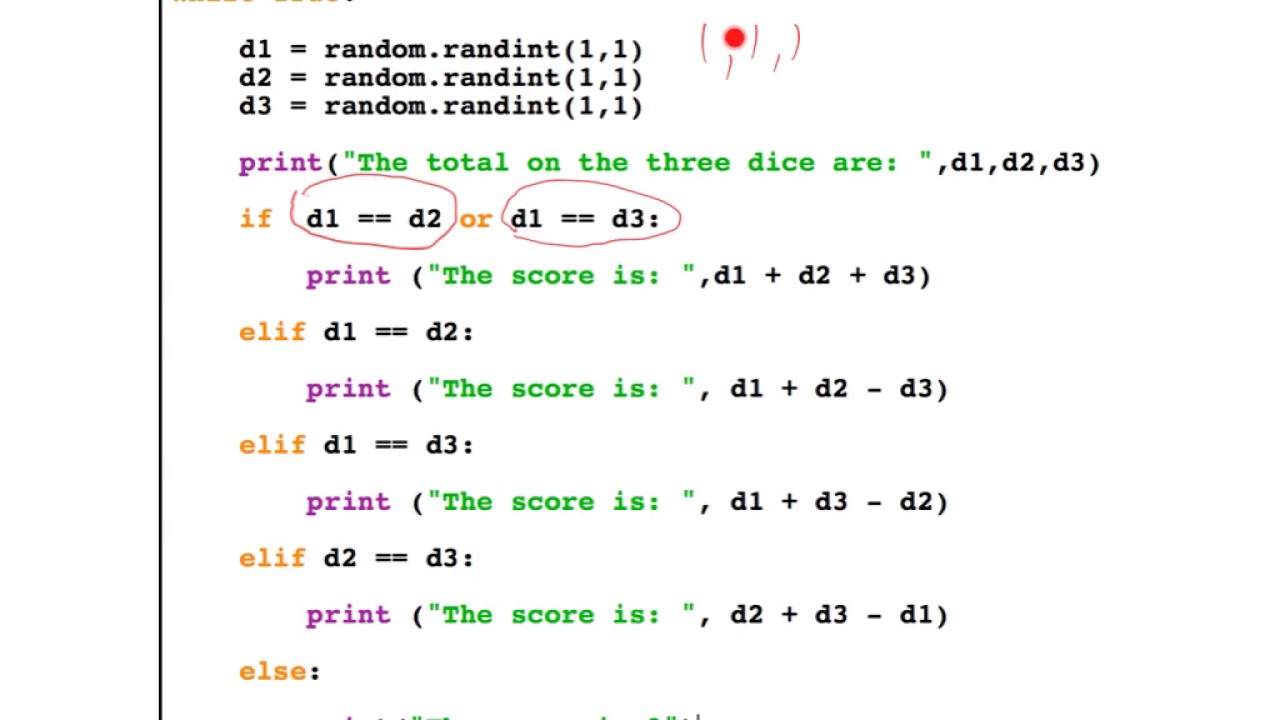